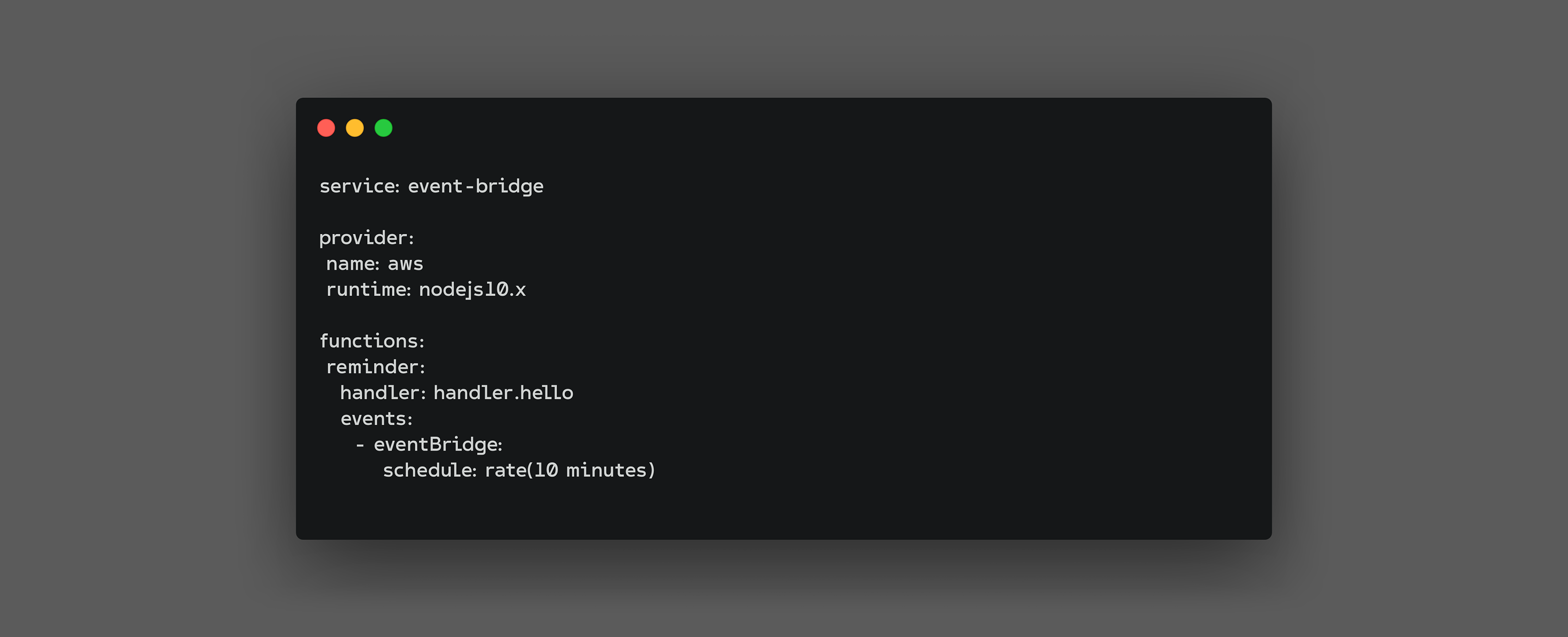
In serverless applications, events are the main communication mechanism. Sources such as API Gateways, databases or data streams emit events while other infrastructure components such as functions react to them in order to fulfill a given business need.
Every major cloud provider has rolled out their own internal event system to reliably dispatch events from component to component within their ecosystem.
Given that events are such an integral piece for serverless architectures, the question arises whether there’s a way to generate and inject custom events into the system. To address this need one pattern which arose throughout the years was the usage of Webhooks to connect external event sources with the cloud. Webhooks are HTTP endpoints which consume HTTP requests, translating the incoming request data into an event which is then forwarded to the respective cloud service.
While this pattern served us well there are a couple of downsides associated with it. Webhooks require additional infrastructure such as API Gateways to be created and maintained. The API endpoint should be scalable and always available to ensure successful event delivery. The act of marshalling and unmarshalling of requests and responses introduces overhead and can be tedious to handle. Scaling the event delivery gets tricky if there’s a need to dispatch events to multiple recipients based on rules. This isn’t the Serverless way.
There’s a clear stance that events in serverless applications should be treated as first-class citizens. It should be easy to create, ingest and react to events. No matter the origin.
Luckily AWS recently announced the EventBridge offering which helps developers build universal, reliable and fully event-driven applications. EventBridge is a serverless pub / sub service which makes it possible to seamlessly connect different event sources with AWS cloud services via event buses. Event publishers can be any (e.g. legacy) application, SaaS providers or internal AWS services. Event consumers can range from EC2 instances, to other event buses to Lambda functions. Given this simplicity, yet flexibility it’s easy to see why other developers call this announcement one of the most important services for serverless application development.
Are you curious how the EventBridge can be used in your serverless applications? The Serverless Framework v1.49.0 has got you covered as it includes native support for the eventBridge
event source!
Let’s take a look into EventBridge and the Serverless Framework eventBridge
event implementation to see how easy it is to build truly event-driven applications without the need for other infrastructure components or Webhook workarounds.
Use Case 1: AWS events
The AWS EventBridge service is internally built on top of CloudWatch Events which makes it possible to easily react to events generated by other AWS services.
Generally speaking there are 2 different types an EventBridge event can be operated with. The "Schedule" type schedules event emission while the "Pattern" type matches incoming event data based on a configured schema to only invoke the Lambda function when the pattern matches.
Let’s start by looking into the "Schedule" type and define an eventBridge
event configuration which invokes our Lambda function every 10 minutes.
In the following serverless.yml
file we declare our reminder
function which has an eventBridge
event source configured to invoke the Lambda function every 10 minutes:
service: event-bridge
provider:
name: aws
runtime: nodejs10.x
functions:
reminder:
handler: handler.hello
events:
- eventBridge:
schedule: rate(10 minutes)
Scheduling the event delivery in that way makes it possible to create 100% serverless cron services (One can even use cron expressions to define when a function should be invoked).
The second, "Pattern" event type gives us great flexibility to react to different events emitted by AWS services such as EC2, Batch, EBS and more. A pattern is basically a schema definition AWS uses to filter out relevant events which are then forwarded to your function.
Let’s imagine that we want to listen to state changes of AWS Key Management Service (KMS). To do that we need to tell AWS that we’re only interested in the KMS service and its event types. The following is a serverless.yml
file set up with such configuration:
service: event-bridge
provider:
name: aws
runtime: nodejs10.x
functions:
kmsNotifier:
handler: handler.hello
events:
- eventBridge:
pattern:
source:
- aws.kms
detail-type:
- KMS Imported Key Material Expiration
- KMS CMK Rotation
- KMS CMK Deletion
More CloudWatch event types and their pattern definitions can be found in the AWS documentation.
If you’re familiar with the Serverless Framework and its different event types you might think that this looks like another way to write schedule
and cloudwatchEvent
event definitions and you’re absolutely right about that. You might remember that we just learned above that the EventBridge service is built on top of CloudWatch Events.
Use Case 2: Custom events
Using internal AWS event sources is nice since it gives us a great flexibility to react to different changes in our infrastructure. However we’ve learned that this capability was already introduced via CloudWatch Events a while back.
What makes EventBridge even more useful is the support for custom events. But how would that work? Every AWS account is preconfigured with an "AWS internal" event bus called default
. This is the bus we’ve just used above when working with internal AWS event sources.
However with the introduction of EventBridge we’re now able to create and configure our own event buses. We can create many different buses for different use cases and / or applications. One could, for example, configure a "marketing" event bus which is solely used for marketing-related event sources and sinks.
Let’s use EventBridge to create our own marketing
event bus. Furthermore let’s see how an external Newsletter application can emit events to that bus to invoke a Lambda function responsible for team-wide notifications.
The following serverless.yml
definition describes a service which manages the notify
function. This function uses the eventBridge
event to create a marketing
event bus and listens to event sources we define as acme.newsletter.campaign
on that event bus:
service: event-bridge
provider:
name: aws
runtime: nodejs10.x
functions:
notify:
handler: handler.hello
events:
- eventBridge:
eventBus: marketing
pattern:
source:
- acme.newsletter.campaign
Next up we need to add some logic to our Newsletter application so that events are emitted to the marketing
event bus everytime something noteworthy happens:
// In our marketing application code
const AWS = require('aws-sdk');
function notifyMarketingTeam(email) {
const eventBridge = new AWS.EventBridge({ region: 'us-east-1' });
return eventBridge.putEvents({
Entries: [
{
EventBusName: 'marketing',
Source: 'acme.newsletter.campaign',
DetailType: 'UserSignUp',
Detail: `{ "E-Mail": "${email}" }`,
},
]
}).promise()
}
if (userHasSubscribed) {
return notifyMarketingTeam(user.email);
}
NOTE: Remember that this Newsletter application is "external", meaning that it can be hosted everywhere. It can even be an old legacy enterprise application you want to integrate with your serverless infrastructure.
Once the code is deployed we’ll reliably receive acme.newsletter.campaign
events on our marketing
event bus which will be forwarded to our notify
Lambda function whenever a new user has subscribed to our newsletter.
This is just one example where custom events and dedicated event buses can be useful. As you can see it’s pretty simple to configure EventBridge and hook it up with existing applications. Following this pattern we can replace pretty much every Webhook based implementation out there. Using EventBridge for such scenarios is more reliable, way easier to setup and scale and cheaper to operate since AWS manages everything for us behind the scenes.
Conclusion
The recent announcement for AWS EventBridge support is a true game changer for serverless application development.
By nature, serverless is all about functions and events. EventBridge provides us with a managed, centralized event bus system we can use to react to any AWS infrastructure event, external SaaS provider events or even our own, custom defined events.
Using the EventBridge as a central component to receive and forward events helps us build reliable, more robust and decoupled event-driven systems at scale.
The Serverless Framework v1.49.0 release ships with first-class citizen support for the evenBridge
event source, making it easy for us to benefit from a serverless event bus system.
Feel free to check our EventBridge docs for more information.
Are you excited about Serverless Framework v1.49.0 and its support for EventBridge? Let us know what you’re planning to build with the Serverless Framework and the eventBridge
event source in the comments below or via Twitter @goserverless.