Want to learn more about Amazon API Gateway HTTP APIs, when it makes sense to use, its limitations and how it compares to other AWS API Gateway APIs? This guide is for you. We'll walk through all the details you need to know to make an informed decision about if you should use AWS HTTP APIs as well as how you can get started with them using the Serverless Framework. We'll also give you several getting started guides and resources if you want to keep learning more.
What are AWS HTTP APIs?
AWS HTTP APIs are the latest iteration of tooling for creating APIs with the Amazon API Gateway. They offer a variety of significant improvements including large reductions in latency and price along with several new integrations. They also lack some of the features you may currently rely on when working with the Amazon API Gateway to build REST APIs.
How do AWS HTTP APIs work?
AWS HTTP APIs are, in many cases, similar to API Gateway REST APIs. They sit between backend services powering your API and all the applications and users making HTTP requests. The Amazon API Gateway HTTP APIs allow interacting with AWS services like AWS Lambda and VPC. It also provides easy authentication with JSON web tokens and many other features that simplify the process of creating HTTP APIs.
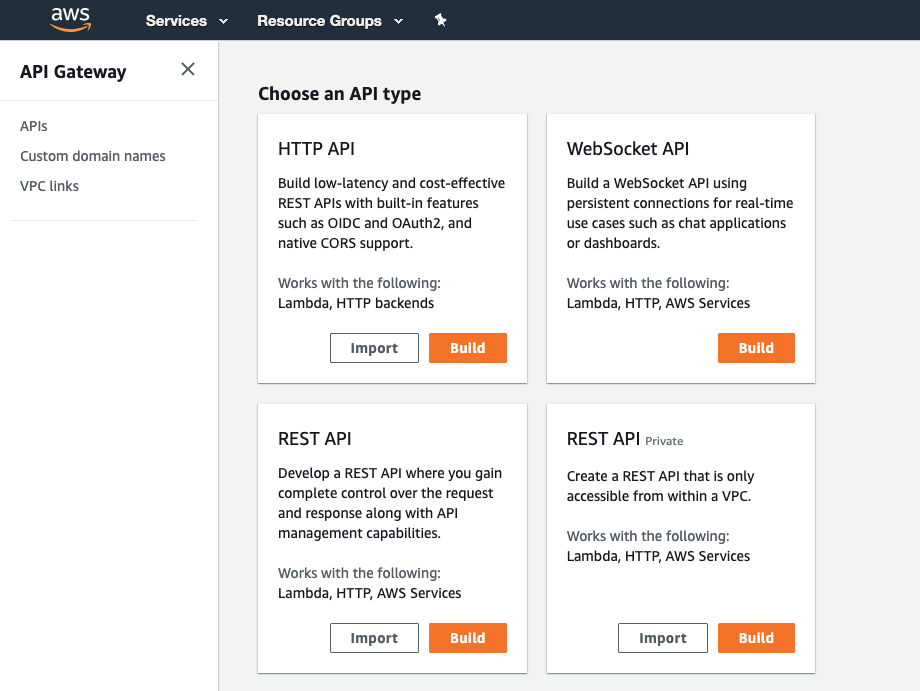
You can create AWS HTTP APIs using the AWS Console or using the AWS CLI, AWS SDKs, or other tooling like the Serverless Framework. The drawback to creating AWS HTTP APIs in the AWS Console is that the structure of those APIs, and changes to them, aren't documented in code. This is why we recommend creating HTTP APIs with the Serverless Framework's HTTP API Event.
What makes AWS HTTP APIs an important tool for Serverless architectures?
AWS HTTP APIs, like API Gateway itself, tie together discrete Lambda Functions and your application's API routes. This allows you to trigger a serverless function response directly to an HTTP request and is a critical component of using serverless APIs within web applications. AWS HTTP APIs also hold several important distinctions over traditional Amazon API Gateway REST APIs ranging from improved developer experience, to improvements in performance and price.
The original Amazon API Gateway product has some barriers to entry for new developers trying to get started with simple HTTP APIs. It required developers to create a variety of different cloud resources to get a single API route setup and connected to a Lambda Function. The new AWS HTTP API portion of the Amazon API Gateway service dramatically simplifies this process and in some cases allows configuring an entire API with a single cloud resource. This also has implications for services managed by CloudFormation, which has limits on the total number of resources per CloudFormation stack.
The pricing and performance of HTTP APIs compared to API Gateway REST APIs is discussed in more detail later, but HTTP API has dramatically reduced both latency and price. These improvements may draw industries that haven't found serverless computing to be an option in the past.
Given the releases of new features for HTTP APIs as of their GA launch in 2020 AWS seems likely to add additional integrations. They added support for VPC endpoints and other integrations, and it seems likely they might continue to add more managed integrations for the HTTP API that simplify the development process even more dramatically.
How do AWS HTTP APIs Integrate with other AWS services?
Let's look at some of the key integrations that HTTP APIs offer with other AWS services ranging from Amazon Cognito to Virtual Private Cloud. Here's a bullet point summary on some of the most notable integrations, followed by more details on each:
- A JSON Web Token integration that makes authentication and authorization easy
- Simplified route integrations and auto-deployed stages
- An integration to connect HTTP APIs to resources inside of a VPC
- The ability to add both HTTP APIs and REST APIs to custom domains
- An integration for adding CORS headers across your API
- Lambda payload and response changes
JWT Authentication and Authorization
In the past, authentication and authorization were supported by AWS Lambda and API Gateway by using custom Lambda authorizers and JWT verification processes. This process involved managing your own Lambda function to process and verify incoming JWTs and then generate an IAM policy that granted it access to your API.
Overall, this was a huge nuisance. You had to extract the JWT from headers, verify that it was formatted correctly and cryptographically validate it with a library like jose (Node.js) or python-jose (Python). After all that, you still had to generate an IAM policy granting access to the API endpoint, and make sure you did it properly to avoid inadvertently caching a policy that denied access to subsequent requests to different endpoints. Essentially, a huge hassle that AWS is offering to take care of for you.
If you're interested in using the JWT integration and the Serverless Framework, you can read more about it here.
Simplified Routes and Deployment Stages
With the new HTTP API, AWS added a simpler method of configuring and deploying API routes and stages.
Essentially, HTTP API routes have an HTTP Method like GET or POST or a catch-all route like ANY. They also have a route or path like /some-path. And they have an integration target such as Lambda or another URI. This makes the overall configuration much simpler. It also appears to be a way for AWS to add more integrations later on, possibly allowing you an easier way to hook into AWS services other than Lambda.
They've also done the same thing with built-in HTTP API stages making them a simpler concept that doesn't require a separate "deployment" resource.
These changes are currently not as significant for Serverless Framework users, as many of these details are already abstracted away when using the Serverless Framework.
VPC Integrations for Private Resources
AWS also recently added the capability to integrate with Private VPC resources like ECS, EKS, and EC2. This means, that your HTTP API (or your old REST APIs), in addition to using Lambda, could hook into other services inside of VPC using an Application Load Balancer, Network Load Balancer, or AWS Cloud Map.
This will be especially useful for organizations that want to provide controlled HTTP access to protected VPC resources. If you already have existing backend resources serving requests, they can now link up with the HTTP or REST APIs as an alternative to something like a public-facing elastic load balancer.
Sharing Custom Domains between REST and HTTP APIs
This addition, allows you to share custom domains between REST and HTTP APIs. This is especially great if you're like to start leveraging the new functionality of HTTP APIs but you're taking it slo moving existing endpoints over. It allows you to start adding new HTTP API endpoints into your current custom domains without having to tear all the old stuff out and move it over immediately.
Say you have an existing REST API running and configured to handle requests related to customer profiles and orders might look like this:
- yourcoolapi.com/customer/{id}/profile
- yourcoolapi.com/customer/{id}/order
Now, you can add in HTTP API endpoints using the same custom domain. So your new endpoints for adding customer subscriptions can still look like:
- yourcoolapi.com/customer/{id}/subscription
But it will be running on the faster, cheaper, HTTP API.
This is one of the most compelling developments in the announcement for organizations that want the cost savings for new development but aren't quite ready to replace existing APIs.
CORS Headers
The new HTTP APIs allow you to easily configure CORS headers to be returned by the API Gateway for every single endpoint of your API instead of in your Lambda handler code. This is pretty snazzy as it lets you change parts of your Lambda code from something like this:
To this:
There is a subset of headers you can control in this way, and the Serverless Framework will help you with this by default. We cover this more in the announcement post of our support for HTTP APIs.
Lambda Payload v2.0
However, that's just half the story. In addition to the global CORS config, there is also a v2.0 Lambda payload coming from API Gateway. This payload is greatly simplified and cuts out a significant portion of the data that was previously available and restructures it. You can review the differences in the AWS Blog post.
This v2.0 payload is optional for HTTP APIs because it may be a breaking change for some existing Lambda functions that rely on data only in the v1.0 format, or that is formatted differently or in a different part of the event payload object.
With the v2.0 payload that API Gateway provides to Lambda, is the option for Lambda to send back an even more simplified response to API Gateway. Previously, Lambda had to return an object with a statusCode and body along with any required headers. But with the addition of the global CORS configuration, the headers were no longer required:
With the v2.0 integration, Lambda can keep sending data back in that format, or it can go a step further and omit a status code entirely and just return the body itself:
This v2.0 integration simplifies the Lambda/API Gateway interaction dramatically and effectively abstracts away the API gateway entirely as you can just return whatever data you'd like and have that sent back via the API. Even better, the v2.0 integration will fall back to a 1.0 integration if it sees the 1.0 integration format (a body and headers).
How do AWS HTTP APIs Work with the Serverless Framework?
You can create your own HTTP APIs with the Serverless Framework in 20 lines of code. Starting with a serverless.yml file:
Along with a very simple get handler inside a hello.py file:
Assuming you've already installed the Serverless Framework, you can then run a serverless deploy and get your first HTTP API endpoint!
If you would like more in-depth guides to HTTP APIs, be sure to check out the Getting Started section below for more examples and tutorials.
Benefits of Amazon API Gateway
There are some notable improvements when using the new HTTP APIs compared to API Gateway REST APIs:
- A cost reduction of ~71% compared to API Gateway REST APIs.
- A reduction of latency by 60% compared to API Gateway REST APIs - this amounts to ~10ms latency or less added by the HTTP API.
Cost Reduction
AWS API Gateway, in the days of only REST APIs, was criticized fairly often for how expensive it was at scale. While it offered a massive set of features, the overall price was pretty steep when you started getting into more significant workloads.
- 100 Million REST API Gateway requests = $350
- 100 Million HTTP API Gateway requests = $100
Upfront, that's a 71% decrease for requests in the 100 Million range.
While REST APIs do have slightly better pricing as your usage scales up, the pricing still stalls at a minimum of $1.51/million requests in us-east-1. This means that the HTTP API will always outperform purely from a request-by-request standpoint. Even at volumes over 20 Billion invocations per month it's still roughly a 40% price reduction.
A few additional consideration here:
- REST APIs do have caching options which may complicate pricing calculations depending on the levels of caching used.
- You may use REST APIs and HTTP APIs differently with other services like AWS Lambda and CloudWatch. These usage differences can have implications for your final bill too.
Reduction of Latency
Another fairly common criticism of API Gateway REST APIs was the additional latency of adding it to your applications. In the past, I haven't seen this as a large motivating factor for avoiding API Gateway. However, for organizations that run very latency-sensitive applications this may be a huge change. According to metrics recently released by AWS, the total p99 or 99th percentile latency added by API Gateway HTTP APIs is now 10ms total - including both the incoming and outgoing request/response processing.
In the past, API Gateway responses via REST APIs sometimes added hundreds of milliseconds of latency. Those numbers were gradually improved on but the criticism remained. It's likely that reductions of up to 60% of the API Gateway portion of the latency may be significant enough to draw interest from new latency-sensitive tech sectors.
This means you may see organizations with latency as a high-priority reconsidering using API Gateway. It also means that those organizations may now find Serverless Application development overall much more appealing as one of the largest challenges they faced before now cut down significantly. As the independent verifications of the new claims of 10ms total latency come in, ad-tech, market-trading or other sectors where latency is critical may start to become more interested in serverless workloads.
What Are the Drawbacks of HTTP APIs?
In addition to all the great new benefits, there's some key differences, limitations and drawbacks of HTTP APIs. Depending on your situation, these could prevent you from using the new HTTP APIs over the older API Gateway REST APIs. AWS has several tables with different support or unsupported features of the HTTP APIs here. So let's take a look at some of the key changes and differences between the two.
When looking at some of these differences I'll include potential workarounds or alternatives:
- No support for usage plans and API Keys
- No wildcard subdomains
- No request/response transformations
- No caching
- No JSON schema validation
- Cannot deploy edge-optimized APIs
- No Amazon X-ray support and limited logging
- Support for catch-all routing
- Deployment, stage, route configuration differences
Usage Plans and API Keys
Usage Plans and API Keys are a great feature of the API Gateway REST APIs. While AWS might add support for this in the future, your best bet is to stick with REST APIs right now and leverage HTTP APIs for any endpoints that don't require usage plans and API Keys.
Wildcard Subdomains
This feature was an excellent option for APIs that needed to provide customers with a branded API and potentially use it to isolate customers' usage.
The options for this now are more limited and would require you to create individual subdomains unless working with the REST APIs exclusively.
No Request/Response Transformations
Using request/response transformations, you could process the incoming or outgoing API Gateway data given a pre-configured transformation template. Currently, your best option are to bring this processing into Lambda or to only use HTTP APIs for endpoints that don't require this transformation.
No Caching
As far as I know, there's no great solution here yet beyond configuring your downstream services to implement their own caching or relying on REST APIs and the caching they offer. Because the HTTP APIs are significantly cheaper and faster caching may not be as important as it was for the REST APIs. You can also always leverage caching to speed up the other parts fo your application. If you use DynamoDB for example you can still leverage DAX.
No JSON Schema Validation
One great option for API Gateway's REST APIs is that you could define the expected payload bodies coming into the API Gateway with JSON Schema Draft 04. We've had an easy way to configure this option in the Serverless Framework too. However, this isn't an option out of the box with HTTP APIs.
The best alternative, and one that many people may already be using is to validate the incoming body directly from the incoming event body when it gets to Lambda. You can use a variety of libraries to do this validation, many of which support JSON Schema already and will allow you to use your existing JSON Schema definitions.
No Edge Optimized or Private APIs
At the moment, you can't have edge optimized or private HTTP APIs. The best best here is to use REST APIs for those you need to be edge optimized or private and HTTP APIs for any others to save on cost.
No Amazon X-ray Support and Limited Logging
For anyone currently relying on Amazon X-ray in their serverless applications, they will need to find an alternative solutions such as Framework Pro to monitor and debug their HTTP API applications. AWS may end up adding X-ray support for HTTP APIs eventually, but at the moment it isn't available.
The same goes for sending access logs to Amazon Kinesis Data Firehose or using API execution logs. At the moment, neither is supported by the HTTP API.
Support for Catch-all Routing
One key difference between AWS HTTP and REST APIs is that HTTP APIs support catch-all routing. This allows you to configure a single endpoint that catches all requests and routes them to a single integration (e.g. a Lambda Function). Our added support for the httpApi event in the Serverless Framework allows you to use this catch-all routing or to configure individual API endpoints how you would normally with the traditional REST API.
Deployment, Stage, Route Configuration Differences
Overall, as we discussed in our initial announcement about Serverless Framework support for HTTP APIs, the underlying API Gateway resources are significantly simplified. Thus far, we've made a few decisions about how to provide you the same Serverless Framework experience you're used to while we allow you to integrate with the HTTP API as a new event type.
The simplicity of discrete stages, easy deployments of those stages, and route/path configurations are still offered to you by the Serverless Framework - both in the REST API and HTTP world. If you see cases where we can add additional functionality or improve the experience in this new world of HTTP APIs please open an issue!
What are AWS HTTP APIs good for?
Your AWS HTTP APIs are a critical part of building serverless APIs. They function as your API server by managing the routes for your API endpoints, connecting custom domains to those routes, and potentially handling authentication for your API. The HTTP APIs will connect every API route to a corresponding Lambda Function or other backend service and handle other requirements like CORS headers or authentication.
With their improvements to latency, they may be suited to some lower-latency requirements, but they may not be the perfect fit for applications that require consistent single or double-digit millisecond latency for all HTTP requests. Additionally, if your application is leveraging GraphQL APIs you many want to consider tools more specifically built for that purpose like AWS AppSync.
AWS HTTP API Limits
As with many AWS services, the default capacity limits can usually be increased with an AWS support request. However, there are some hard limitations to be aware of when using HTTP APIs. Here are a few of those:
A 30 seconds integration timeout
This means that you must either resolve an integration request within 30 seconds or HTTP APIs will timeout. However, you can use something like Lambda Event Destinations in order to quickly accept requests coming in from HTTP APIs, without waiting on a response from the backing resource (e.g. Lambda).
10 MB Payload size
Every HTTP APIs can only process up to a 10 MB payload. Anything beyond that will trigger an error. For APIs that require submitting images or larger files however, there are many design patterns that you can leverage in order to process and accept images. Using pre-signed S3 URLs is one of the most common methods for this.
1500 ms Timeouts for auth endpoints
Because HTTP APIs offer a managed integration with JSON Web Key Set (JWKS) and OpenID Connect discovery endpoints, there is also a default timeout if those endpoints do not respond within 1.5 seconds. This means that your endpoint provider, such as Auth0, Okta, or others, will need to respond to HTTP requests within that time.
Other Limitations
There are other limitations when using HTTP APIs, but many of them require an AWS support request to increase. For a full and updated list of current limitations to HTTP APIs you can review this table of limitations.
WS HTTP API Pricing
As with many AWS services, the pricing for AWS HTTP APIs differs by AWS region. For this price guide, I will be using the latest numbers for the US East (Northern Virginia) Region.
The HTTP APIs come with a 12-month free tier that includes one million API calls per month for up to 12 months. Outside of that free tier, or after the 12-month period has ended, HTTP API requests are charged at a rate of $1.00 for every million requests for the first 300 million requests per month.
If you're doing more than 300 million requests per month, the price for those additional requests then drops to $0.90 per million requests.
Let's look at a few examples that assume you're past the 12 month AWS free tier period:
Assume you have a REST API with 100 customers, each customer makes 100,000 HTTP API requests per month on average. In this scenario, you can model your requests and the price for your requests like this:
- Total requests: 100 x 100,000 = 10,000,000 requests/month
- Total cost: 10 Million x $1.00/1 Million requests = $10.00/month.
Let's say you have a surge of new clients and a surge of requests per client as your product grows. Let's imagine you now have 2,000 customers and they're making, on average, 300,000 HTTP API requests per month.
- Total requests: 2,000 x 300,000 = 600,000,000 requests/month
- Total cost for first 300 Million requests: 300 Million * $1.00/1 Million requests = $300
- Total cost for the second 300 Million requests: 300M * $0.9/1 Million requests = $270
- Total cost: $570
Now remember, this is only related to the HTTP API service itself. If you're using other services like AWS Lambda to process requests, S3 to store files, or DynamoDB to store data records, all of those will have their own billing models. You can review our Lambda cost calculations or DynamoDB pricing sections of other guides to see how that might impact your bottom line.
Critically, you'll want to recognize the major differences between HTTP API pricing and the pricing for other API Gateway services.
Getting Started
We're collecting guides, tutorials and examples around the AWS HTTP API - and we'd love to feature yours! Don't hesitate to reach out to us if you think you've found or written a great one yourself so we can feature it here:
- Serverless Framework Support for AWS HTTP APIs - Our announcement on our support for AWS HTTP APIs
- Serverless Auth with AWS HTTP APIs - A guide to using the JWT integration with the Serverless Framework and new HTTP APIs
- Your next AWS HTTP API Guide!
Hopefully this gives you a good overview on the significance of the changes and differences between AWS HTTP APIs and REST APIs. In the future, you can look out for our upcoming "Official Guide to AWS HTTP APIs" where we'll take you through the process of developing with HTTP APIs. We'll also update that guide with any new developments that may arise in the coming months as AWS adds to the functionality of HTTP APIs.